react router 설치
D:\springboot2\bootspring\src\main\webapp\shop
npm install react-router-dom
✅ 알아두쇼
- App.js는 하나만 있어야 함!~
- react안에는 jsp,jstl 코드는 사용 불가! (html 형태에만 가능)
- ES14 => 15
암호화 기능 추가 (특수문자 형태로)
원자 (waitSync) -> 다중 스레드
[A,B] => [A~B] 계산 가능
[A&&B] : 교집합
[A - [0-9]] : 중첩데이터 확인 ex) 023459034 -> 259
..... 등ㅎ - Linux에 설치 세팅
Linux 서버 -> nodejs 설치 => Linux 사용자 디렉토리 => React npx 설치 => npm Router 설치
1. yum install nodejs , yun install npm
2. npm install -g yarn
3. npx install create-react-app 사용자디렉토리
4. 사용자 디렉토리로 이동
5. npm install react-router-dom
6. npm start 또는 yarn start - react 에서는 @Controller 사용 X => @RestController 사용함!
👀 useEffect , useState / fetch + oracle
- index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import { BrowserRouter } from 'react-router-dom';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<BrowserRouter>
<App />
</BrowserRouter>
);
- App.js
import React,{} from 'react'
import { Route, Routes } from 'react-router-dom'
import Idcheck from './Idcheck' //상대경로 => src의 실제 js파일 찾을 때 사용(.js 작성 안해도 됨)
//export하기 위한 함수
function App(){
return(
<>
<Routes>
<Route path='/Idcheck' element={<Idcheck />}></Route>
</Routes>
</>
);
}
export default App;
- idcheck.js
back에서 ok or no로 값 받아와서 체크
import React,{ useEffect, useState } from 'react';
export default function Idcheck(){
//react의 key배열 - java의 new와 같은 형태로 객체와 시키는 형태구조
var [test,testval] = useState('');
//useEffect : 즉시 실행 이벤트 핸들링 함수
useEffect(function(){ //useEffect(()=>{ }); 요렇게 해도됨
testval('키 배열에 값을 입력해보기')
console.log("이벤트 핸들링 전용 함수 사용")
});
function mid_check(){ //onclick 함수
var mid = document.getElementById("mid");
if(mid.value == ""){
alert("아이디를 입력해주세요.");
mid.focus();
}else{
//API통신 Ajax => fetch , axios(터미널로 설치해야함)
fetch('/api/idcheck',{
method : "POST",
headers : {
'content-type' : 'application/x-www-form-urlencoded'
},
body : new URLSearchParams({id : mid.value})
}).then(function(result_data){
return result_data.text();
}).then(function(result_api){
if(result_api == "ok"){
alert("사용가능한 아이디입니다.")
}else if(result_api=="no"){
alert("이미 사용중인 아이디입니다.")
mid.value="";
mid.focus();
}
}).catch(function(error){
console.log(error);
});
}
}
return(
<>
{test}
아이디 : <input type="text" name="mid" id="mid" /><br />
<input type="button" value="중복체크" onClick={mid_check} />
</>
);
}
1. defalut : 변수형을 지정하지 않고 함수를 그대로 반영시 사용하는 명령어
2. useEffect : 이벤트 핸들러를 기본으로 하는 함수 (즉시 실행되는 이벤트 핸들링 함수)
useState : 키 배열을 선언하는 함수 (원시배열)
=> 이 두개 백엔드가 많이씀
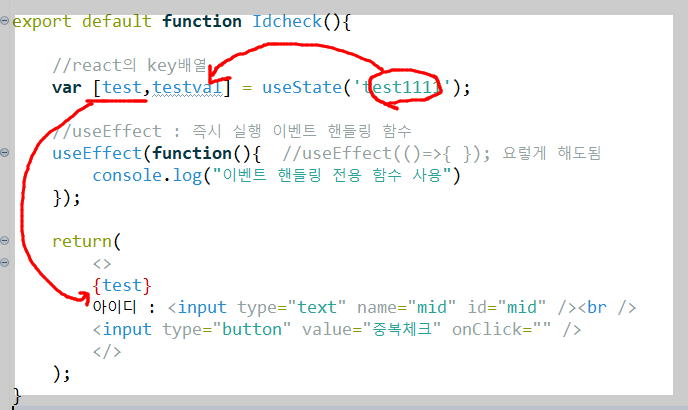
3. API통신 Ajax => fetch , axios(터미널로 설치해야함)
back-end
- restapi.java (controller)
react 에서는 @Controller 사용 X => @RestController 사용함!
package kr.co.sen;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import jakarta.annotation.Resource;
@RestController //api 전용 controller
public class restapi {
//api는 무조건 결과값을 전달해줘야함 - 해당 값을 받을 변수 미리 선언
private String result = null;
//Dto
@Resource(name="customer_dto")
customer_dto customer_dto;
//Service Interface
@Autowired
api_member_service api_member_service;
//API 전용 Controller이므로 CrossOrigin 활용 필쑤
//(React 포트 추가 , * 로 해도 됨 - class 밖에 써도 됨)
@CrossOrigin(origins="localhost:3000", allowedHeaders = "*")
@PostMapping("/api/idcheck")
public String idcheck(@RequestParam(value="id" , required=false) String id) {
try {
List<customer_dto> one = api_member_service.onedata(id);
if(one.size() > 0) { //사용중인 아이디가 있는 경우
this.result = "no";
}else { //없는경우 - 사용가능
this.result = "ok";
}
}catch(Exception e) {
this.result = "error";
}
return this.result;
}
}
JSON In Java » 20240303
implementation group: 'org.json', name: 'json', version: '20240303'
JSON.simple » 1.1.1
implementation group: 'cohttp://m.googlecode.json-simple', name: 'json-simple', version: '1.1.1'
=> gradle 의존성 추가함
https://dev-eunse.tistory.com/310 여기에서 썼었음 - dto는 여기서 만든거 사용
-cms_dto.java
package kr.co.sen;
import org.springframework.stereotype.Repository;
import lombok.Data;
import lombok.Getter;
import lombok.Setter;
@Data
@Repository("customer_dto")
public class customer_dto {
Integer c_idx;
String c_id,c_name,c_hp,c_email,c_tel,c_post;
String c_road,c_address,c_level,c_area,c_join;
}
- api_mapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="kr.co.sen.api_member_repo">
<select id="onedata" resultType="kr.co.sen.customer_dto" parameterType="String">
select * from CUSTOMER where c_id=#{c_id}
</select>
</mapper>
- api_member_repo.java (interface)
package kr.co.sen;
import java.util.List;
import org.apache.ibatis.annotations.Mapper;
@Mapper
public interface api_member_repo {
//아이디 중복체크 및 개인정보 확인시 사용
List<customer_dto> onedata(String c_id);
}
- api_member_service.java (interface)
package kr.co.sen;
import java.util.List;
public interface api_member_service {
List<customer_dto> onedata(String c_id);
}
- api_member_impl.java (class)
package kr.co.sen;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class api_member_impl implements api_member_service{
@Autowired
private api_member_repo apirepo;
@Override
public List<customer_dto> onedata(String c_id) {
List<customer_dto> one = apirepo.onedata(c_id);
return one;
}
}
👀 export (idcheck.js ..) - App.js에서 import함
import React,{} from 'react'
import { Route, Routes } from 'react-router-dom'
import { idcheck } from './idcheck' //상대경로 => src의 실제 js파일 찾을 때 사용(.js 작성 안해도 됨)
/*export 방식 1 */
export let idcheck = function(){
return(
<>
123
</>
);
}
// App.js에서 import {Idcheck} from './Idcheck' 요렇게 import할 때
/*방식 2 */
//export하기 위한 함수
function idcheck(){
return(
<>
123
</>
);
}
export default idcheck;
/* 방식 3 */
export default function Idcheck(){
return(
<>
123
</>
);
}
// 방식 2,3은 App.js에서 import Idcheck from './Idcheck' 요렇게 {}없이 import할 때
// defalut : 변수형을 지정하지 않고 함수를 그대로 반영시 사용하는 명령어
'CLASS > REACT' 카테고리의 다른 글
#2-3 / React - 회원가입 리스트 및 페이징,검색 (data select) (1) | 2024.09.13 |
---|---|
#2-2 / React - 회원가입 (data insert , React + 주소 api) (0) | 2024.09.10 |
#1-3 / STS React Setting (0) | 2024.09.09 |
#1-2 / React Router,Link (0) | 2024.09.09 |
#1-1 / React Setting (1) | 2024.09.09 |