[ 알아야함 ]
- ip or 도메인주소
- Port 정보
포트 중복사용 불가 - 단, TCP와 UDP가 구분될 경우 가능
(cmd로 열려있는지 닫혀있는지 확인 netstat -an) - TCP : 외부 연결 프로토콜 (전송속도 빠름)
- UDP : 내부 연결 프로토콜 (전송속도 느리나 1:1일 경우 TCP보다 빠름)
[ network ]
예외처리 필수
Inet4Address,Inet6Address : ipv4,ipv6 ( InetAddress : 부모 , 무조건 엄마먼저 써야함) -> new 안씀
⚡ ip 확인
//getByName : 접속할 도메인 또는 ip 주소명 출력 InetAddress ia = Inet4Address.getByName("localhost"); System.out.println(ia); //localhost/127.0.0.1 System.out.println(ia.getHostName()); //localhost / 도메인명만 출력 System.out.println(ia.getHostAddress());//127.0.0.1 / ip만 출력 //getAllByName : 접속할 도메인에 관련된 ip 모둔 주소 리스트 출력 => 배열로 받아야함 InetAddress all[] = Inet4Address.getAllByName("naver.com"); System.out.println(Arrays.asList(all)); //네이버에 연결되어있는 서버 다 들어옴
⚡ socket 열기
socket : 외부에서 접속을 할 수 있도록 오픈하는 하나의 경로
ServerSocket : 서버를 오픈하는 라이브러리 (소켓번호를 세팅)
InetSocketAddress : (도메인 또는 ip , 오픈할 포트번호)
getRemoteSocketAddress : 상대방의 ip정보를 확인 및 접속 소켓 확인
accept() : 접속 //다른 누군가 접속해야 뜸 , (무한반복문 안에 작성시 계속 열려있게 가능)
bind() : 집어넣겟다..?
public static void main(String[] args) { try { //ServerSocket : 서버를 오픈하는 라이브러리 (소켓번호를 세팅) //InetSocketAddress : (도메인 또는 ip , 오픈할 포트번호) ServerSocket ss = new ServerSocket(); //cmd ipconfig 내 ip확인후 기입 , "localhost" 가능 InetSocketAddress ia = new InetSocketAddress("172.30.1.83", 7070); /* bind : add,append 처럼 ss라는 소켓에 ia를 집어넣겠다 정적 바인딩 : 자식 생성 후 호출 동적 바인딩 : 부모에게 접근하는 형태 */ ss.bind(ia); //구축함 (동적 바인딩) System.out.println("연결중입니다...."); //소켓 오픈시 해당 라인에서 정지(대기상태) //소켓으로 접속시 아래의 코드 활성화 Socket sc = ss.accept(); //accept : 접속 //getRemoteSocketAddress : 상대방의 ip정보를 확인 및 접속 소켓 확인 InetSocketAddress ia2 = (InetSocketAddress)sc.getRemoteSocketAddress(); System.out.println("연결 확인!"); }catch(Exception e) { System.out.println("해당 서버에 문제가 발생하였습니다"); } }
InetSocketAddress : 오픈할때 , InetAddress : 가져올때
▼
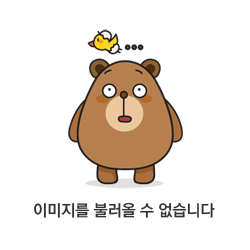
⚡ 외부 서버에 있는 이미지를 다운로드 받는 코드
URL u = new URL(url); //url 경로 확인(라이브러리)
URLConnection con = u.openConnection(); //해당 서버로 접근
InputStream is = u.openStream(); //openStream : 외부에서 접속하는 파일 형태(앞엔 무조건 엄마)
public static void main(String[] args) { try { Scanner sc = new Scanner(System.in); System.out.println("웹에서 가져올 이미지 주소를 입력하세요 : "); String url = sc.nextLine(); URL u = new URL(url); //url 경로 확인(라이브러리) URLConnection con = u.openConnection(); //해당 서버로 접근 //int imgsize = con.getContentLength(); //파일 사이즈 확인 //String imgtype = con.getContentType(); //파일 속성 및 확장자 확인 long date = con.getDate(); //해당 파일에 접속한 날짜 확인 SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd-mmss"); //unix 시간을 라이브러리를 통해 현재 시간 형태로 변환(이건 oop) String today = sdf.format(date); //-- img를 가져옴 - stream //openStream : 외부에서 접속하는 파일 형태(앞엔 무조건 엄마) InputStream is = u.openStream(); BufferedInputStream bs = new BufferedInputStream(is); byte b[] = new byte[bs.available()]; //-- pc에 저장(저장 경로 및 파일명 -> 저장) String copy = "D:\\"; FileOutputStream fs = new FileOutputStream(copy + today + ".jpeg"); int size = 0; while((size = bs.read(b)) !=-1 ) { fs.write(b,0,size); // 파일 크기에 맞춰서 저장형태 fs.flush(); } fs.close(); bs.close(); is.close(); System.out.println("해당 파일을 정상적으로 다운로드 완료했습니다"); }catch(Exception e) { e.printStackTrace(); System.out.println("해당 서버로 접속이 제한되었습니다."); } }
'CLASS > JAVA' 카테고리의 다른 글
#17 / remind3 (0) | 2024.05.24 |
---|---|
#16-2 / 서버 - TCP (0) | 2024.05.23 |
#15-3 / csv 데이터 저장 (0) | 2024.05.22 |
#15-2 / StreamWriter,StreamReader (0) | 2024.05.22 |
#15-1 / 이미지(binary) (0) | 2024.05.22 |